If a requirement comes your way to programmatically update the validators that are present on your Episerver Forms element(s), the snippets below will help!
Validators are registered in a slightly peculiarly way – it’s a single string property, which contains a delimited list of the validator type names:
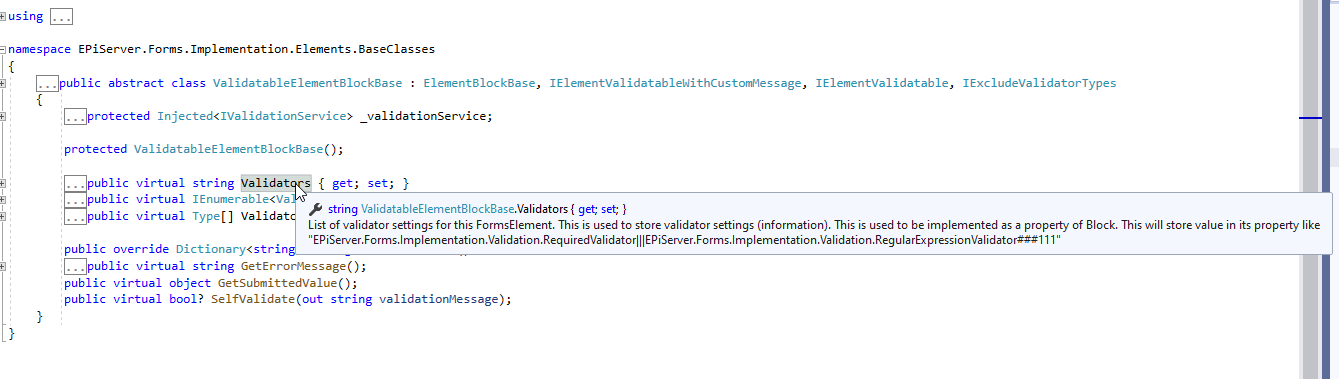
The delimiter does have a strongly-typed constant to represent it – which is found here:
EPiServer.Forms.Constants.RecordSeparator
In addition, validator error messages are represented by a list of Key/Value pairs – the key being the validator type name, and the value being the error message.
Method to update a form element’s validator
public void ApplyValidatorToFormElement<T>(InputElementBlockBase formElement, string errorMessage= "", string extra= "") where T : ElementValidatorBase { var formElementWritable = formElement.CreateWritableClone() as InputElementBlockBase; var formElementValidators = formElementWritable.Validators ?? string.Empty; var validators = new string[] { typeof(T).FullName + extra, formElementValidators }; if (!string.IsNullOrEmpty(errorMessage)) { // Strip out any existing validator messages of this type first var newValidatorMessages = formElementWritable.ValidatorMessages.Where(x => x.Validator != typeof(T).FullName) .ToList(); newValidatorMessages.Add(new ValidatorMessage { Validator = typeof(T).FullName, Message = errorMessage }); formElementWritable.ValidatorMessages = newValidatorMessages; } formElementWritable.Validators = string.Join(EPiServer.Forms.Constants.RecordSeparator, validators); contentRepository.Save((IContent)formElementWritable, SaveAction.Publish, AccessLevel.NoAccess); }
Example
ApplyValidatorToFormElement<RequiredValidator>(formElement, "This field is required");
Regular Expressions
These are slightly more funky – as the Regex pattern is embedded into the validator name with a delimiter. The below method can help achieve this:
public void ApplyRegularExpressionValidatorToFormElement(InputElementBlockBase formElement, Regex regularExpression, string errorMessage) { var regexFormat = $"##{regularExpression.ToString()}###{regularExpression.ToString()}"; ApplyValidatorToFormElement<RegularExpressionValidator>(formElement, errorMessage, regexFormat); }
Example
ApplyRegularExpressionValidatorToFormElement(formElementBlock, new Regex("^[a-zA-Z0-9 '-]+$"), "Text fields can only consist of letters or numbers");
Happy coding!